This walkthrough shows you how to create unbound hierarchical WebGrid.
During this walkthrough, you will learn how to do the following:
- Use WebGridTable.
- Use WebGridRow.
Prerequisites
In order to complete this walkthrough, you will need the following:
- Visual Studio 2005/2008/2010 Application.
Step-By-Step Instructions
- Launch Visual Studio.NET 2008.
- Click on File menu, then select New and click Project.
- Select Visual C# Project in Project Types.
- Select ASP.NET Web Application in the Template box.
- Specify the Project's Location and click OK.
- Drag WebGrid instance into WebForm.
- Right click on WebGrid instance and choose WebGrid.NET Designer.
- Go to Advanced tab - RootTable, set DataMember and Caption to Authors.
- Checked Table Header Visible checkbox in Basic Properties expandable panel.
- Focus to Columns and add 3 columns (AuthorID, LastName and FirstName).
- In ChildTables add one table and name it "Books".
- Checked Table Header Visible checkbox in Basic Properties expandable panel.
- Focus to Columns and add 4 columns (TitleID, Title, Type, and Price) to this table.
- Set the Price's DataType to "System.Double" and DataFormatString to "c".
- In Page_Load server side event, add these codes to setup RootTable and the ChildTable
C# | ![]() |
protected void Page_Load(object sender, EventArgs e) { body.Style.Add("overflow", "hidden"); /* setup root rows and add them to RootTable */ WebGridRow[] rootRows = new WebGridRow[6]; for (int i = 0; i <= 6; i++) rootRows[i] = WebGrid1.RootTable.CreateRow(); rootRows[0].Cells.SetItemData(new string[] { "172-32-1176", "White", "Johnson" }); rootRows[1].Cells.SetItemData(new string[] { "213-46-8915", "Green", "Marjorie" }); rootRows[2].Cells.SetItemData(new string[] { "238-95-7766", "Carson", "Cheryl" }); rootRows[3].Cells.SetItemData(new string[] { "267-41-2394", "O'Leary", "Michael" }); rootRows[4].Cells.SetItemData(new string[] { "274-80-9391", "Straight", "Dean" }); rootRows[5].Cells.SetItemData(new string[] { "341-22-1782", "Smith", "Meander" }); WebGrid1.RootTable.Rows.AddRange(rootRows); /* - create child rows and add them to the parent row in the RootTable * - in this sample, the second row in the root is not expandable * - first row is expanded programmatically * - all expandable rows should have at least one child row and the SetHierarchicalType() * must be set to the Children collection. */ WebGridTable childTable = WebGrid1.RootTable.ChildTables[0]; WebGridRow[] childRows = new WebGridRow[11]; for (int i = 0; i < 11; i++) childRows[i] = childTable.CreateRow(); childRows[0].Cells.SetItemData(new object[] { "BU1032", "The Busy Executive's Database Guide", "Business", 19.99 }); childRows[1].Cells.SetItemData(new object[] { "BU1111", "Cooking with Computers: Surreptitious Balance Sheets", "Business", 11.95 }); childRows[2].Cells.SetItemData(new object[] { "BU2075", "You Can Combat Computer Stress!", "Business", 2.99 }); childRows[3].Cells.SetItemData(new object[] { "BU7832", "Straight Talk About Computers", "Business", 19.99 }); childRows[4].Cells.SetItemData(new object[] { "MC2222", "Silicon Valley Gastronomic Treats", "Cooking", 19.99 }); childRows[5].Cells.SetItemData(new object[] { "MC3021", "The Gourmet Microwave", "Cooking", 14.95 }); childRows[6].Cells.SetItemData(new object[] { "MC3026", "The Psychology of Computer Cooking", "Undecided", 10.29 }); childRows[7].Cells.SetItemData(new object[] { "PC1035", "But Is It User Friendly?", "Popular Computer", 11.05 }); childRows[8].Cells.SetItemData(new object[] { "PC8888", "Secrets of Silicon Valley", "Popular Computer", 24.99 }); childRows[9].Cells.SetItemData(new object[] { "PC9999", "Net Etiquette", "Popular Computer", 9.49 }); childRows[10].Cells.SetItemData(new object[] { "PS1372", "Computer Phobic AND Non-Phobic Individuals: Behavior Variations", "Psychology", 14.69 }); rootRows[0].Children.SetHierarchicalType(); rootRows[0].Children.AddRange(new WebGridRow[] { childRows[0], childRows[1], childRows[2] }); rootRows[0].ExpandChildRow(); rootRows[1].ChildNotExpandable = true; rootRows[2].Children.SetHierarchicalType(); rootRows[2].Children.AddRange(new WebGridRow[] { childRows[3], childRows[4] }); rootRows[3].Children.SetHierarchicalType(); rootRows[3].Children.AddRange(new WebGridRow[] { childRows[5], childRows[6] }); rootRows[4].Children.SetHierarchicalType(); rootRows[4].Children.AddRange(new WebGridRow[] { childRows[7], childRows[8] }); rootRows[5].Children.SetHierarchicalType(); rootRows[5].Children.AddRange(new WebGridRow[] { childRows[9], childRows[10] }); } |
Here is the result :
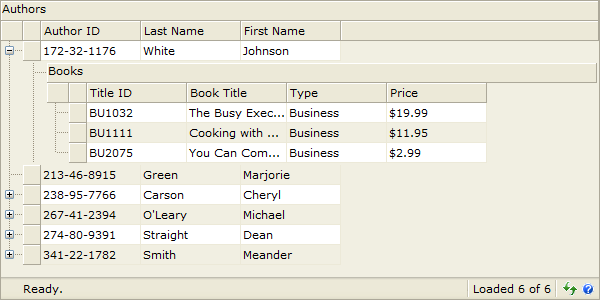