WebScheduler has tight integration with WebCallOut (part of WebDesktop.NET®). By
using WebCallOut, the information of each location of the resources can be shown.
It uses CustomTemplate with FlyPostBack technology to retrieve the information from
server-side. This topic will show you how to integrate WebScheduler with WebCallOut.
To integrate with WebCallOut
- Drag WebScheduler and WebCallOut controls to the WebForm.
- Create a custom resource using OnTimelineCustomResource WebScheduler's
client-side events. In the following code, it will create resource and location
template.
function WebScheduler1_OnTimelineCustomResource(controlId, resourcesId)
{
var WebScheduler1 = ISGetObject(controlId);
var labelHeader1 = WebScheduler1.ClientFindResourceCustomControl("Header", "Label1");
var labelHeader2 = WebScheduler1.ClientFindResourceCustomControl("Header", "Label2");
labelHeader1.innerText = "Resource";
labelHeader2.innerText = "Location";
var resources = WebScheduler1.Resources;
for (var i = 0; i < resourcesId.length; i++)
{
var label1 = WebScheduler1.ClientFindResourceCustomControl(resourcesId[i], "Label1");
var label2 = WebScheduler1.ClientFindResourceCustomControl(resourcesId[i], "Label2");
for (var j = 0; j < resources.length; j++)
{
if (resources[j].Resourceid == resourcesId[i])
{
label1.innertext = resources[j].ResourceName;
label2.innerText = resources[j].Location;
break;
}
}
}
return true;
}
|
- Create the following table in WebCallOut's content template.
<ContentTemplate>
<table style="width: 100%; height: 100%;" cellspacing="0" cellpadding="0">
<tbody>
<tr>
<td style="vertical-align: middle; padding-right: 5px;">
<asp:Image ID="imgPicture" runat="server" Width="50px" BorderColor="Black" BorderStyle="Solid"
BorderWidth="1px" Height="40px" />
</td>
<td>
<table style="width: 100%; height: 100%; font-family: Segoe UI; font-size: 8pt;"
cellspacing="0" cellpadding="0">
<tr>
<td style="width: 60px;">
Country
</td>
<td>
:<asp:Label ID="lblCountry" runat="server" Text="Label"></asp:Label>
</td>
</tr>
<tr>
<td style="width: 60px;">
Capital
</td>
<td>
:<asp:Label ID="lblCapital" runat="server" Text="Label"></asp:Label>
<tr>
<td style="width: 60px;">
Time zone
</td>
<td>
:<asp:Label ID="lblTimeZone" runat="server" Text="Label"></asp:Label>
</td>
</tr>
</table>
</td>
</tr>
</tbody>
</table>
</ContentTemplate>
|
- Use WebScheduler's Show server-side event to provide the information
in WebCallOut when OnTheFlyPostBack is invoked.
protected void WebCallOut1_Show(object sender, ISNet.WebUI.WebDesktop.WebCallOutEventDataArgs e)
{
string selectedControlText = Request.Form["selectedControlText"].ToString();
switch (selectedControlText)
{
case "London":
imgPicture.ImageUrl = "./Images/england.jpg";
lblCountry.Text = "England";
lblCapital.Text = "London";
lblTimeZone.Text = "GMT (UTC0)";
break;
case "Toronto":
imgPicture.ImageUrl = "./Images/canada.jpg";
lblCountry.Text = "Canada";
lblCapital.Text = "Ottawa";
lblTimeZone.Text = "Eastern (EST)(UTC-5)";
break;
case "Milan":
imgPicture.ImageUrl = "./Images/italy.jpg";
lblCountry.Text = "Italy";
lblCapital.Text = "Rome";
lblTimeZone.Text = "CET (UTC+1)";
break;
}
}
|
- Create WebCallOut's OnBeforeShow client-side event to invoke OnTheFlyPostBack,
so that WebCallOut will retrieve the information from server-side.
function WebCallOut1_OnBeforeShow(controlId)
{
var WebCallOut1 = ISGetObject(controlId);
var selectedControl = WebCallOut1.TargetControlElement;
WebCallOut1.AddInput("selectedControlText", selectedControl.innerText);
return true;
}
|
- Create ShowWebCallOut and HideWebCallOut methods
to show/hide WebCallOut.
function ShowWebCallOut(labelScheduler)
{
var callOut = ISGetObject("WebCallOut1");
var targetId = document.getElementById(labelScheduler.id);
if (targetId.innerText != "Location")
{
callOut.TargetControlId = targetId.id;
window.setTimeout(function() { callOut.ShowAutoDetect(targetId, true); }, 10);
}
}
function HideCallOut(labelScheduler)
{
var callOut = ISGetObject("WebCallOut1");
callOut.Hide();
}
|
- Finally, invoke ShowWebCallOut() and HideWebCallOut()
in ResourcesContentTemplate like following.
<ResourcesContentTemplate>
<table cellpadding="0" cellspacing="0" style="padding: 2 2 2 2; width: 100%; height: 100%;
font-family: Segoe UI; font-size: 8pt;">
<tr>
<td style="border-right: solid 1px #9199a4; width: 50%;">
<asp:Label ID="Label1" runat="server" Text="Resource Name"></asp:Label>
</td>
<td>
<asp:Label ID="Label2" runat="server" Text="Location" onmouseover="return
ShowWebCallOut(this)"
onmouseout="return HideCallOut(this)"></asp:Label>
</td>
</tr>
</table>
</ResourcesContentTemplate>
|
- Run the project. The WebCallOut will appear with location's information when user
hover to London, Toronto or Milan.
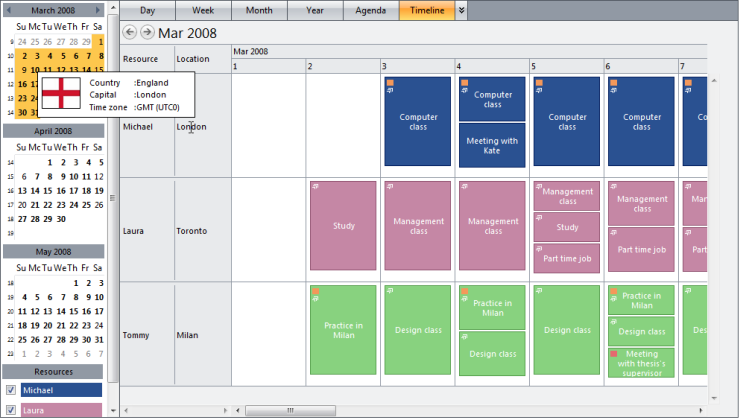