You can set load on demand feature in WebTreeView for better performance.
This topic will show you how to implement the above scenario.
To set load on demand in WebTreeView (Basic)
- Right click on WebTreeView instance and choose Properties
to open its Properties window.
- Set the EnableLoadOnDemand property to True.
- Run the project.
You can add TreeViewNodes based on retrieved data. This scenario can only be done
in unbound TreeView mode.
This topic will show you how to implement the above scenario.
To add TreeViewNodes based on retrieved data (Advanced Load On Demand scenario)
- Right click on WebTreeView and choose Properties to open its Properties
window.
- Set EnableLoadOnDemand to True.
- Create a dataset and named it dsNorthwind. This
dataset will be used to get data from database and the data will be added
to WebTreeView.
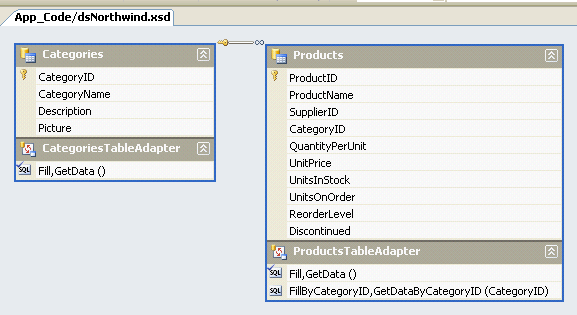
- Right click on WebTreeView control and choose WebTreeView.NET Designer.
Switch to Tree Editor tab and create a parent node in the
WebTreeView.
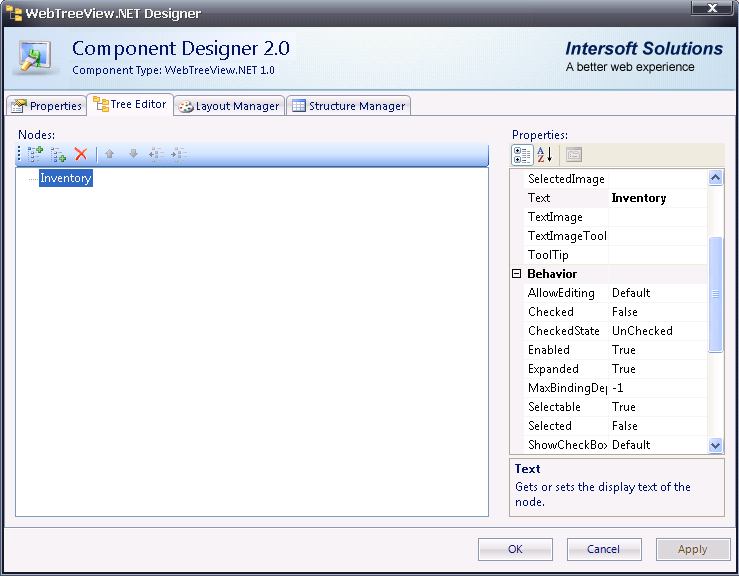
- Implement WebTreeView's InitializeChildNodes server side event.
protected void WebTreeView1_InitializeChildNodes(object sender, ISNet.WebUI.WebTreeView.WebTreeViewNodeEventArgs e)
{
PopulateNode(e.Node);
}
private void PopulateNode(WebTreeViewNode node)
{
// Call the appropriate method to populate a node at a particular level.
switch (node.Depth)
{
case 0:
// Populate the first-level nodes.
PopulateCategories(node);
break;
case 1:
// Populate the second-level nodes.
PopulateProducts(node);
break;
default:
// Do nothing.
break;
}
}
private void PopulateCategories(WebTreeViewNode node)
{
// Query for the product categories. These are the values
// for the second-level nodes.
dsNorthwindTableAdapters.CategoriesTableAdapter adapter = new dsNorthwindTableAdapters.CategoriesTableAdapter();
dsNorthwind.CategoriesDataTable table = adapter.GetData();
// Create the second-level nodes.
if (table.Count > 0)
{
// Iterate through and create a new node for each row in the query results.
// Notice that the query results are stored in the table of the DataSet.
foreach (dsNorthwind.CategoriesRow row in table.Rows)
{
WebTreeViewNode newNode = new WebTreeViewNode();
newNode.Name = "Category" + row.CategoryID.ToString();
newNode.Text = row.CategoryName;
newNode.Value = row.CategoryID;
node.Nodes.Add(newNode);
}
}
}
private void PopulateProducts(WebTreeViewNode node)
{
// Query for the products of the current category. These are the values
// for the third-level nodes.
dsNorthwindTableAdapters.ProductsTableAdapter adapter = new dsNorthwindTableAdapters.ProductsTableAdapter();
dsNorthwind.ProductsDataTable table = adapter.GetDataByCategoryID((int)node.Value);
// Create the second-level nodes.
if (table.Count > 0)
{
// Iterate through and create a new node for each row in the query results.
// Notice that the query results are stored in the table of the DataSet.
foreach (dsNorthwind.ProductsRow row in table.Rows)
{
WebTreeViewNode newNode = new WebTreeViewNode();
newNode.Name = "Product" + row.ProductID.ToString();
newNode.Text = row.ProductName;
newNode.Value = row.ProductID;
node.Nodes.Add(newNode);
}
}
}
protected void WebTreeView1_InitializeNode(object sender, WebTreeViewNodeEventArgs e)
{
if (e.Node.Depth == 2)
e.Node.ChildNodeExpandable = false;
}
|
- Run the sample and you will get a result like screenshot below.
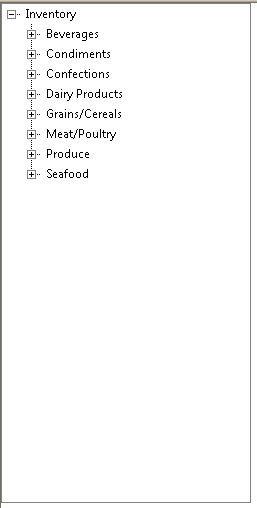